OpenCV - YOLO Video Detection
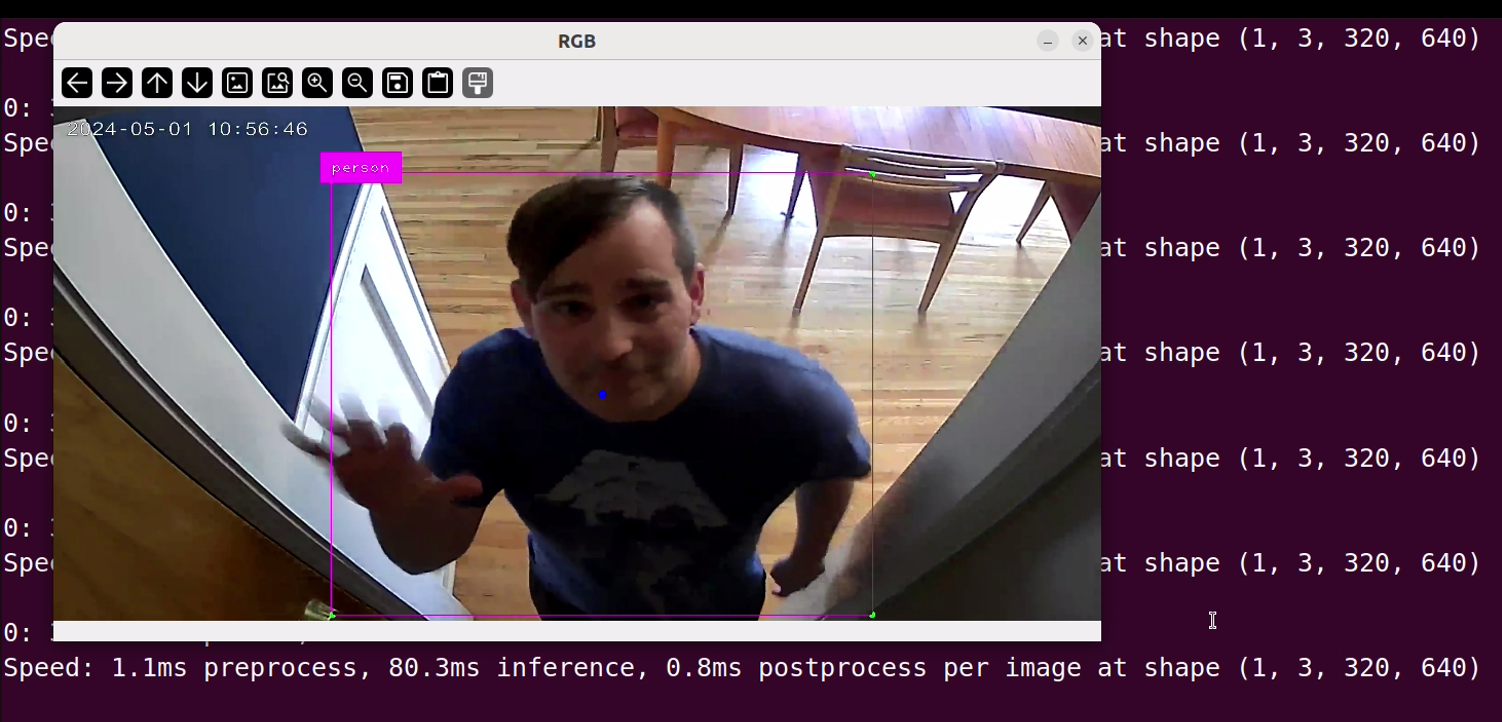
OpenCV is an open source computer vision library with many different algorithms for detection and classification.
YOLO is one of the best and efficient video detection libraries. The code below used OpenCV and YOLO for people detection.
import cv2
import pandas as pd
from ultralytics import YOLO
import cvzone
import numpy as np
#use only 'person' detection in yolo model
model=YOLO('best.pt')
model.classes = [0]
def RGB(event, x, y, flags, param):
if event == cv2.EVENT_MOUSEMOVE :
point = [x, y]
print(point)
cv2.namedWindow('RGB')
cv2.setMouseCallback('RGB', RGB)
#Video capture from PC camera
cap = cv2.VideoCapture(0)
assert cap.isOpened()
my_file = open("coco.txt", "r")
data = my_file.read()
class_list = data.split("\n")
area1=[(544,12),(587,377),(713,372),(643,13)]
area2=[(763,17),(969,343),(1016,298),(924,16)]
while True:
ret,frame = cap.read()
if not ret:
cap.set(cv2.CAP_PROP_POS_FRAMES, 0)
continue
frame=cv2.resize(frame,(1280,720))
#Run prediction only on 'person'
results=model.predict(frame, classes = [0])
a=results[0].boxes.data
px=pd.DataFrame(a).astype("float")
for index,row in px.iterrows():
x1=int(row[0])
y1=int(row[1])
x2=int(row[2])
y2=int(row[3])
d=int(row[5])
c=class_list[d]
cx=int(x1+x2)//2
cy=int(y1+y2)//2
w,h=x2-x1,y2-y1
cvzone.cornerRect(frame,(x1,y1,w,h),3,2)
cv2.circle(frame,(cx,cy),4,(255,0,0),-1)
cvzone.putTextRect(frame,f'person',(x1,y1),1,1)
cv2.imshow("RGB", frame)
if cv2.waitKey(1)&0xFF==27:
break
cap.release()
cv2.destroyAllWindows()
What's my age again?
Example below uses OpenCV for gender and age detection. Code is based on reference below
https://github.com/smahesh29/Gender-and-Age-Detection